Managing application state in modern web development has always been a challenging yet essential task. Angular developers, in particular, have relied on state management solutions like NgRx to streamline their workflows. With the introduction of SignalStore in Angular 17, developers now have a more lightweight and efficient tool for managing application state. In this article, we will delve into the power of @ngrx/signalstore: a deep dive into task management, exploring its core features, benefits, and practical applications in building a scalable task management system.
What Is SignalStore?
SignalStore, a feature of the NgRx ecosystem, leverages signals to simplify state management. Signals are reactive variables that automatically update components when their values change. This eliminates the complexity of observables and subscriptions, offering a more straightforward approach to handling state in Angular applications.
Why Choose SignalStore for Task Management?
SignalStore shines in scenarios requiring dynamic state updates, such as task management applications. Here are its standout features:
- Simplicity: It reduces boilerplate code, making state management more intuitive.
- Performance: SignalStore ensures efficient rendering even in large-scale applications with frequent updates.
- Extensibility: It integrates seamlessly with other libraries and supports custom extensions.
- Error Handling: Built-in mechanisms make error detection and recovery easier.
By understanding these advantages, developers can harness the power of @ngrx/signalstore: a deep dive into task management to create responsive and reliable applications.
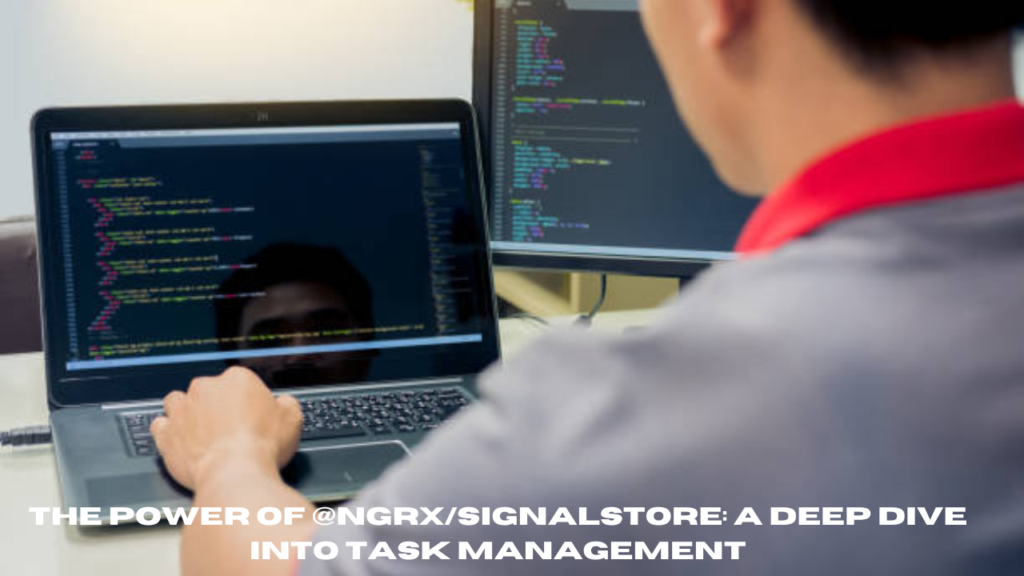
Building a Task Management Application with SignalStore
Step 1: Setting Up the Project
To get started, create a new Angular project using the Angular CLI:
ng new task-manager
Install the SignalStore package:
ng add @ngrx/signal-store
Step 2: Define the Task Model
Define a Task interface in TypeScript to represent individual tasks:
interface Task {
id: number;
title: string;
completed: boolean;
}
Step 3: Create the SignalStore
Use the createSignal
function to manage the state:
import { createSignal } from '@ngrx/signal';
const tasksSignal = createSignal<Task[]>([]);
const newTaskTitleSignal = createSignal('');
Step 4: Implement Task Management Logic
Create functions to handle common task operations:
- Add Task:
function addTask() {
const newTask: Task = {
id: Date.now(),
title: newTaskTitleSignal(),
completed: false,
};
tasksSignal.update((tasks) => [...tasks, newTask]);
newTaskTitleSignal.set('');
}
- Toggle Completion:
function toggleTaskCompletion(taskId: number) {
tasksSignal.update((tasks) =>
tasks.map((task) =>
task.id === taskId ? { ...task, completed: !task.completed } : task
)
);
}
- Filter Tasks:
function filterTasks(filter: 'all' | 'active' | 'completed') {
tasksSignal.update((tasks) => {
if (filter === 'active') return tasks.filter((task) => !task.completed);
if (filter === 'completed') return tasks.filter((task) => task.completed);
return tasks;
});
}
Step 5: Connect SignalStore to Components
Subscribe to signals in your Angular components:
import { Component } from '@angular/core';
import { tasksSignal, newTaskTitleSignal, addTask, toggleTaskCompletion } from './task-store';
@Component({
selector: 'app-task-list',
template: `
<div>
<input [(ngModel)]="newTaskTitle$" placeholder="Add a new task" />
<button (click)="addTask()">Add Task</button>
<ul>
<li *ngFor="let task of tasks$">
<input type="checkbox" [(ngModel)]="task.completed" (change)="toggleCompletion(task.id)" />
{{ task.title }}
</li>
</ul>
</div>
`,
})
export class TaskListComponent {
tasks$ = tasksSignal();
newTaskTitle$ = newTaskTitleSignal();
addTask() {
addTask();
}
toggleCompletion(taskId: number) {
toggleTaskCompletion(taskId);
}
}
Advanced Features
- Data Persistence: Save tasks to local storage or a backend API to maintain state across sessions.
- Error Handling: Implement try-catch blocks and validation to handle potential issues.
- Testing: Use unit tests to validate SignalStore logic and ensure application reliability.
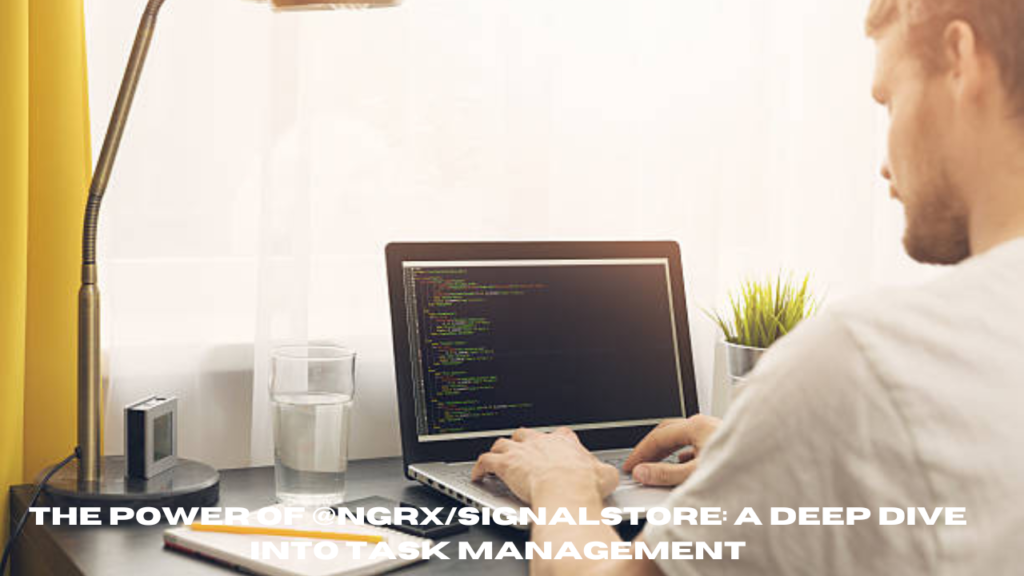
Also read: Exploring rewdrbll.com: A Comprehensive Review
Last Reviews
SignalStore revolutionizes Angular state management by combining simplicity, performance, and flexibility. Whether you are building a basic task manager or a complex enterprise application, leveraging the power of @ngrx/signalstore: a deep dive into task management will enhance your development experience and deliver robust, maintainable solutions.